Building Simple Web API Service Adapters in .NET
No doubt that the introduction of REST API revolutionized the technology landscape in a short span of time. It triggered the metamorphosis in the information exchange systems, especially the transition from Web Services to Web APIs in the software development. In the .NET world, WCF still got its place considering the support for multiple transport protocols, encodings, transactions, message security and exchange patterns. But Web API is an open source light-weight HTTP only framework which supports wide variety of media types with a broad range of clients including browsers, mobiles, tablets, IoT devices etc.
But for those early migrators of Web APIs, a major hindrance was the extra hardship on parsing and reading the xml or json response manually. No need to ask the component creators how much they might have missed the Web API equivalent of WSDL proxy creation in .NET projects. Life was so easier with that ‘Add Web Reference’ proxy creation tool within the Visual Studio IDE or the command line wsdl.exe utility. Consequently, they had to go through the hassle of manual response processing. And that was exactly the reason, why back in those days, I had no choice other than to create our own simple adapter for connecting clients to REST API handling all the cumbersome tasks under the hood.
Time has changed and now there are many solutions available on the market based on the structured metadata for OData integrated APIs. Swagger based on Open API standard provides some client generator tools. If we are creating the public REST API, we can rely on swagger and make use of it to publish your service interface. Even though to use that within the same project or domain, is definitely an overkill. And more importantly, if the service response data classes change frequently during the development and the client need to update the code accordingly, then using our own light weight adapter is efficient in terms of time and effort.
How It Works:
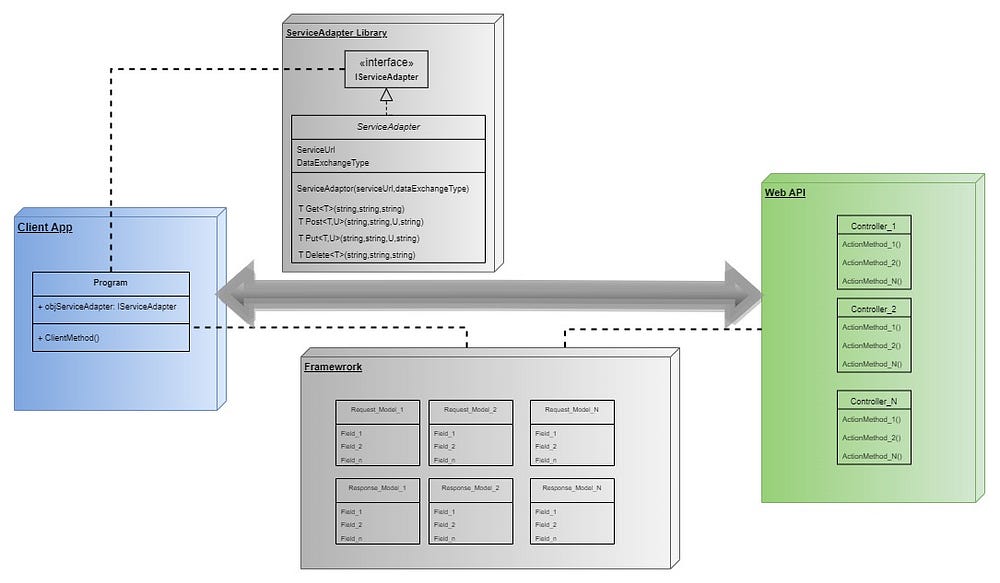
We need to move the shared request and response data model classes from WebAPI to a framework class library project and share the framework assembly with the clients. And then the client initializes the ServiceAdapter utility with the API endpoint to connect to the WebAPI. Later it calls the required POST or GET generic methods using response and request types defined in the framework assembly. Also, the controller and action names are passed as parameters (building from Enum values would be a safe option to avoid typo errors).
Query string also can be passed as one of the param if needed. For POST method calls, request body data is passed as object to the ServiceAdapter Post<T,U>(…) function. The respective GET or POST methods returns the response object directly back to the client. Client is not concerned about the connection logic or serialization/deserialization mechanism other than passing required object models and getting the response object back.
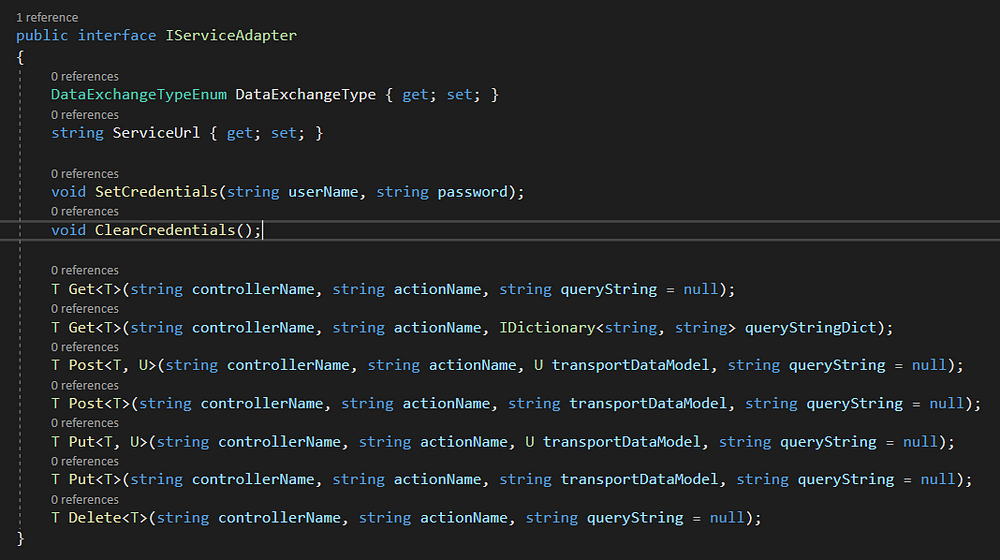
The sample ServiceAdapter demo created using VSCode contains:
- ServiceAdapter: The main reusable library used for API connectivity
- Framework: The transport data model definitions for demo
- WebAPI: A WebAPI project created for demo
- TestConsole: An API client created as console app for testing ServiceAdapter
Check the complete solution here:
You can't perform that action at this time. You signed in with another tab or window. You signed out in another tab or…github.com
For the past half decade, there have been many industry standard solutions available in the market, to publish the REST API Services which can be used to generate client connectors as well.
Swagger and Swashbuckle
Meanwhile, Swashbuckle is an open source project within WebAPI that are built with Microsoft ASP.NET Core. This includes three core components namely SwaggerGen, SwaggerUI and Swagger. Nugets packages need to be installed, if not installed already, to create swagger documents and customize the content and endpoints.
With well-defined data annotations and xml comments in the code, swashbuckle auto generates OpenAPI JSON document. Document endpoint can be configured within the code with various options.
builder.Services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new() { Title = "Sanee Codes Web API", Version = "v1" });
});
......
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "Sanee Codes Web API"));
WADL vs WSDL
Web Application Definition Language aka WADL is supposed to be the REST counterpart of WSDL (Web Service Definition Language). But this is an area where lot of hot debates going on and its standardization is still pending.
WSDL is an XML notation describing a SOAP based web service by specifying the location of the service and its methods. This is used by the client to create the proxy for requests and defines the interface provided by the web service.
On the other hand, WADL is a light weight XML vocabulary defining the RESTful services using a set of resource elements which contains param and method elements. While request element specifies the input representation and required types, the response element defines the service response and the fault information if any.
RAML
RESTful API Modeling Language (RAML) defines the specification for REST API using YAML scripts. This is a powerful language as a contract for APIs which allows teams to define, build and collaborate on APIs with less effort and time.
RAML is both machine and human readable language making it easily editable with minimal technical knowledge. Tools like RAML/API Design, API Console and the API Notebook assist developers to model an API in a concise and reusable way which makes it easy to manage the API lifecycle from design to deployment.
RAML is an open source language with tools and parsers for common language features that support structured files and inheritance that address cross-cutting concerns. API gateways like MuleSoft, AWS API Gateway, Apigee, Restlet and Akana currently supporting RAML and further API gateways can be benefited by converting RAML specification to OpenAPI.
Open API Specification
Formerly known as Swagger Specification, the OpenAPI Specification is a specification for machine-readable interface definition language for defining, creating, consuming and visualizing RESTful web services. Being language-agnostic, with OpenAPI’s declarative resource specification, clients can understand and consume services without knowledge of server code.
Open Data Protocol
OData enables the creation and consumption of REST APIs, which allow clients to publish and edit resources, identified using URLs and data models, using simple HTTP messages. OData builds on HTTP, AtomPub and JSON and uses the HTTP verbs to indicate the operation on the resources which are GET, POST, PUT, PATCH and DELETE. OData endpoint URL can contain query options which can be used to filter, order, map and paginate data. Ex: OData/Employees?$orderby=Name&$filter=Age lt 40
Related Topics of Interest:
Resource Description Framework (RDF)
RESTful Service Description Language (RSDL)
Hypermedia as the Engine of Application State (HATEOAS)
GData (Google Data Protocol)
GraphQL
Final Words:
Many of the above listed language and specifications should be used while creating public APIs and also for creating APIs for clients who wanted to manage their own client creation and documentation. But for internal REST APIs which needs to be used within same project or domain, using our own simple solution like the ServiceAdapter library explained at the beginning, would be a viable solution. This light weight client helps to manage the data model changes across multiple teams efficiently and effortlessly within an organization.
Keep it thin and simple for our own people at least :)
Links
Complete source code is available on the Git Repository.
GitHub: https://github.com/mailsanish/ServiceAdapter
Medium: https://medium.com/@sanish.abraham/building-simple-web-api-service-adapters-in-net-72df98620d83
LinkedIn: https://www.linkedin.com/in/sanishabraham/
Keywords:
Web API, Rest API, Service Adapter, Service Connector, Open API, OData, WADL, WSDL, Swagger, Swashbuckle, RAML, Open Data Protocol, RDF, RSDL, HATEOAS, GData, Google Data Protocol, GraphQL, .NET, .NET Core, C#, Visual Studio Code, API Connectivity, SwaggerGen, SwaggerUI, RESTful
HashTags:
#webapi #restapi #serviceadapter #serviceconnector #openapi #odata, #wadl #wsdl #swagger #swashbuckle #gdata #apiconnectivity #raml #vscode #apiadapter #apiconnector #dotnet #csharp #netcore #graphql #swaggerui #swaggergen #hateoas #rsdl #opendata #restful #api #rest
Comments
Post a Comment